客户端如何从本地数据库取数据
- 编程技术
- 2025-01-29 00:27:44
- 1
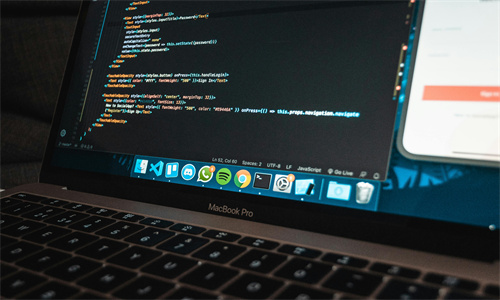
客户端从本地数据库取数据通常涉及以下步骤:1. 选择数据库:首先需要确定客户端将使用哪种数据库,例如SQLite、MySQL、PostgreSQL等。2. 数据库连接:...
客户端从本地数据库取数据通常涉及以下步骤:
1. 选择数据库:首先需要确定客户端将使用哪种数据库,例如SQLite、MySQL、PostgreSQL等。
2. 数据库连接:客户端需要建立与本地数据库的连接。以下是几种常见数据库的连接方法:
SQLite:可以使用Python的`sqlite3`模块连接SQLite数据库。
```python
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
```
MySQL:可以使用`mysql-connector-python`或`pymysql`等库连接MySQL数据库。
```python
import mysql.connector
conn = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="mydatabase"
)
cursor = conn.cursor()
```
PostgreSQL:可以使用`psycopg2`库连接PostgreSQL数据库。
```python
import psycopg2
conn = psycopg2.connect(
dbname="mydatabase",
user="myuser",
password="mypassword",
host="localhost"
)
cursor = conn.cursor()
```
3. 查询数据:使用SQL语句查询所需的数据。
```python
cursor.execute("SELECT FROM mytable")
```
4. 获取数据:将查询结果提取到客户端程序中。
```python
rows = cursor.fetchall()
for row in rows:
print(row)
```
5. 关闭连接:操作完成后,关闭数据库连接。
```python
cursor.close()
conn.close()
```
以下是一个简单的示例,演示如何使用Python和SQLite从本地数据库获取数据:
```python
import sqlite3
连接到SQLite数据库
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
执行SQL查询
cursor.execute("SELECT FROM mytable")
获取查询结果
rows = cursor.fetchall()
打印查询结果
for row in rows:
print(row)
关闭连接
cursor.close()
conn.close()
```
请根据你使用的数据库类型和编程语言调整上述代码。
本文链接:http://www.xinin56.com/bian/378849.html
上一篇:深圳特控线和一本线的区别
下一篇:移动硬盘如何挂载