有源代码如何修改class
- 编程技术
- 2025-02-06 00:55:00
- 1
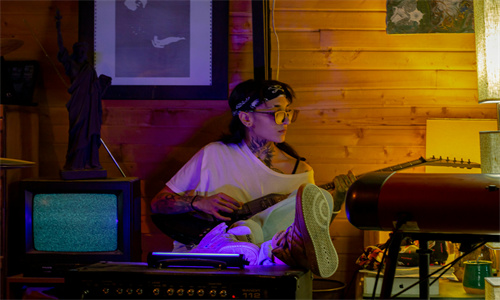
要修改一个类的代码,你需要根据你想要改变的具体方面来进行。以下是一些常见的修改类的场景和相应的代码示例: 1. 修改类的属性```pythonclass MyClass...
要修改一个类的代码,你需要根据你想要改变的具体方面来进行。以下是一些常见的修改类的场景和相应的代码示例:
1. 修改类的属性
```python
class MyClass:
def __init__(self, attribute):
self.attribute = attribute
修改类属性
class MyClass:
def __init__(self, attribute):
self.new_attribute = attribute 修改属性名称
self.attribute = 123 修改属性值
```
2. 修改类的方法
```python
class MyClass:
def my_method(self):
return "Original method"
修改类方法
class MyClass:
def my_method(self):
return "Modified method"
```
3. 添加新的属性或方法
```python
class MyClass:
def __init__(self, attribute):
self.attribute = attribute
添加新的属性
class MyClass:
def __init__(self, attribute, new_attribute):
self.attribute = attribute
self.new_attribute = new_attribute
添加新的方法
class MyClass:
def __init__(self, attribute):
self.attribute = attribute
def new_method(self):
return "This is a new method"
```
4. 修改类的继承
```python
class ParentClass:
def parent_method(self):
return "Parent method"
修改子类继承
class MyClass(ParentClass):
def __init__(self, attribute):
super().__init__()
self.attribute = attribute
如果要改变继承的父类
class MyClass(AnotherParentClass):
def __init__(self, attribute):
super().__init__()
self.attribute = attribute
```
5. 修改类的装饰器
```python
def my_decorator(func):
def wrapper():
print("Before function execution")
func()
print("After function execution")
return wrapper
class MyClass:
@my_decorator
def my_method(self):
print("This is my method")
修改装饰器
class MyClass:
@my_decorator
def my_method(self):
print("This is my method, modified")
```
在修改类的时候,确保你的修改不会破坏类的现有行为,除非这是你的目的。修改类时,通常需要考虑代码的可维护性和向后兼容性。如果你在修改一个已经广泛使用的类,那么确保你有一个好的理由和充分的测试来确保更改不会引入新的问题。
本文链接:http://www.xinin56.com/bian/481311.html