如何用方向键移动控制光标的速度和距离
- 编程技术
- 2025-02-08 22:00:42
- 1
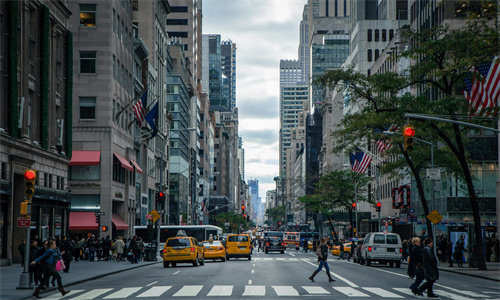
要使用方向键来控制光标的速度和距离,你可以通过编程来实现这个功能。以下是一个简单的示例,使用Python的Tkinter库来创建一个窗口,并通过方向键来控制光标(在这个...
要使用方向键来控制光标的速度和距离,你可以通过编程来实现这个功能。以下是一个简单的示例,使用Python的Tkinter库来创建一个窗口,并通过方向键来控制光标(在这个例子中是一个小方块)的移动速度和距离。
```python
import tkinter as tk
class MovingCursorApp:
def __init__(self, root):
self.root = root
self.root.title("Directional Cursor Movement")
创建一个画布
self.canvas = tk.Canvas(root, width=400, height=400, bg='white')
self.canvas.pack()
创建一个光标
self.cursor = self.canvas.create_rectangle(10, 10, 20, 20, fill='blue')
设置初始速度和距离
self.speed = 5
self.distance = 0
绑定方向键
self.root.bind("
self.root.bind("
self.root.bind("
self.root.bind("
def move_up(self, event):
self.distance += self.speed
self.canvas.move(self.cursor, 0, -self.speed)
def move_down(self, event):
self.distance += self.speed
self.canvas.move(self.cursor, 0, self.speed)
def move_left(self, event):
self.distance += self.speed
self.canvas.move(self.cursor, -self.speed, 0)
def move_right(self, event):
self.distance += self.speed
self.canvas.move(self.cursor, self.speed, 0)
创建窗口并运行应用
root = tk.Tk()
app = MovingCursorApp(root)
root.mainloop()
```
这段代码创建了一个窗口,其中包含一个画布和一个蓝色的光标。通过按下方向键,你可以控制光标的移动。每次按下方向键,光标都会以一定的速度(在这个例子中是5个像素)移动,直到它到达画布的边缘。
这个例子使用了Tkinter库,它是Python的标准GUI库,不需要安装额外的包。你可以根据自己的需求调整速度和距离。如果你想要实现更复杂的逻辑,比如限制光标只能在画布内移动,你可以添加额外的条件检查来确保光标不会移动到画布的外面。
本文链接:http://xinin56.com/bian/525781.html