python如何打包
- 编程技术
- 2025-02-06 07:38:40
- 1
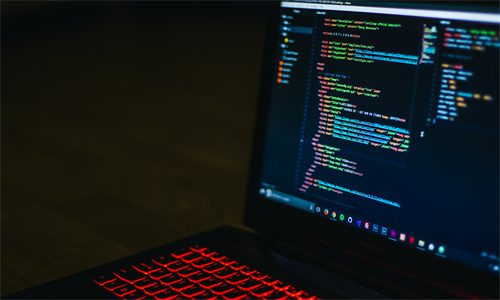
在Python中,打包通常指的是将Python应用程序打包成一个可执行文件或安装包,以便可以在没有Python环境的系统上运行。以下是一些常用的方法: 1. 使用PyI...
在Python中,打包通常指的是将Python应用程序打包成一个可执行文件或安装包,以便可以在没有Python环境的系统上运行。以下是一些常用的方法:
1. 使用PyInstaller
PyInstaller是一个可以将Python脚本转换为独立可执行文件的工具。
安装PyInstaller:
```bash
pip install pyinstaller
```
打包Python脚本:
```bash
pyinstaller --onefile your_script.py
```
这会将`your_script.py`打包成一个单独的可执行文件。
2. 使用cx_Freeze
cx_Freeze也是一个用于创建Windows、MacOSX和Linux的可执行文件的打包工具。
安装cx_Freeze:
```bash
pip install cx_Freeze
```
创建setup.py文件:
```python
from cx_Freeze import setup, Executable
setup(
name = "your_script",
version = "0.1",
description = "A simple script",
executables = [Executable("your_script.py")]
)
```
打包:
```bash
python setup.py build
```
3. 使用Py2exe
Py2exe是专门为Windows系统设计的,可以将Python脚本转换为Windows可执行文件。
安装Py2exe:
```bash
pip install py2exe
```
创建setup.py文件:
```python
from distutils.core import setup
import py2exe
setup(console=['your_script.py'])
```
打包:
```bash
python setup.py py2exe
```
4. 使用PyOxidizer
PyOxidizer是一个现代的打包工具,可以用于创建多种操作系统和架构的可执行文件。
安装PyOxidizer:
```bash
pip install pyoxidizer
```
创建打包脚本:
```python
from oxidizer import Builder
创建Builder实例
builder = Builder()
添加Python脚本
builder.add_python_script("your_script.py")
配置打包选项
builder.set_script_name("your_script")
builder.set_entry_point("main")
构建可执行文件
builder.build()
```
注意:
打包过程中,确保所有依赖的库都已经被安装。
打包后的可执行文件可能会因为缺少系统依赖而无法运行。
希望这些信息能帮助你!如果你有更具体的需求,可以进一步提问。
本文链接:http://xinin56.com/bian/486742.html
上一篇:高中选科有哪些科目
下一篇:岭南大学是985还是211