如何用vb画斜线
- 编程技术
- 2025-01-31 15:19:34
- 1
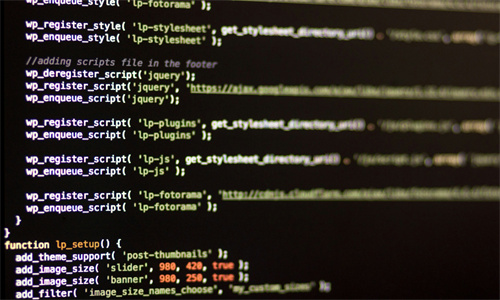
在Visual Basic(VB)中,你可以使用多种方法来绘制斜线。以下是一些常见的方法: 使用 `Line` 方法你可以使用 `Line` 方法来绘制直线,包括斜线。...
在Visual Basic(VB)中,你可以使用多种方法来绘制斜线。以下是一些常见的方法:
使用 `Line` 方法
你可以使用 `Line` 方法来绘制直线,包括斜线。`Line` 方法需要两个坐标点来定义直线的起点和终点。
```vb
Private Sub DrawLine()
' 创建画布
Dim pictureBox As New PictureBox()
pictureBox.Width = 300
pictureBox.Height = 300
pictureBox.BorderStyle = BorderStyle.FixedSingle
' 绘制斜线
pictureBox.Paint += New PaintEventHandler(AddressOf pictureBox_Paint)
' 显示画布
Me.Controls.Add(pictureBox)
' 绘制斜线
Private Sub pictureBox_Paint(sender As Object, e As PaintEventArgs)
Dim g As Graphics = e.Graphics
g.DrawLine(Pens.Black, 0, 0, pictureBox.Width, pictureBox.Height)
End Sub
End Sub
```
使用 `Graphics` 对象
你也可以使用 `Graphics` 对象来绘制斜线,这提供了更多的控制。
```vb
Private Sub DrawLine()
' 创建画布
Dim pictureBox As New PictureBox()
pictureBox.Width = 300
pictureBox.Height = 300
pictureBox.BorderStyle = BorderStyle.FixedSingle
' 绘制斜线
pictureBox.Paint += New PaintEventHandler(AddressOf pictureBox_Paint)
' 显示画布
Me.Controls.Add(pictureBox)
' 绘制斜线
Private Sub pictureBox_Paint(sender As Object, e As PaintEventArgs)
Dim g As Graphics = e.Graphics
Dim pen As New Pen(Color.Black)
Dim angle As Single = 45 ' 斜率
Dim width As Integer = pictureBox.Width
Dim height As Integer = pictureBox.Height
Dim x1 As Integer = 0
Dim y1 As Integer = 0
Dim x2 As Integer = width
Dim y2 As Integer = height
' 计算斜线方向
Dim slope As Single = Tan(angle)
Dim dx As Integer = (x2 x1) slope
Dim dy As Integer = (y2 y1) slope
' 绘制斜线
g.DrawLine(pen, x1, y1, x1 + dx, y1 + dy)
End Sub
End Sub
```
以上代码演示了如何在VB中绘制斜线。你可以根据需要调整斜率和线条的颜色。
本文链接:http://xinin56.com/bian/409391.html
上一篇:本田思域1.5T豪华版上路多少线
下一篇:pscs6自动保存文件在哪里找